Building an Intelligent Stock Assistant with pydantic-ai and Groq LLM
Implementation of a type-safe stock price assistant leveraging LLM capabilities and real-time market data
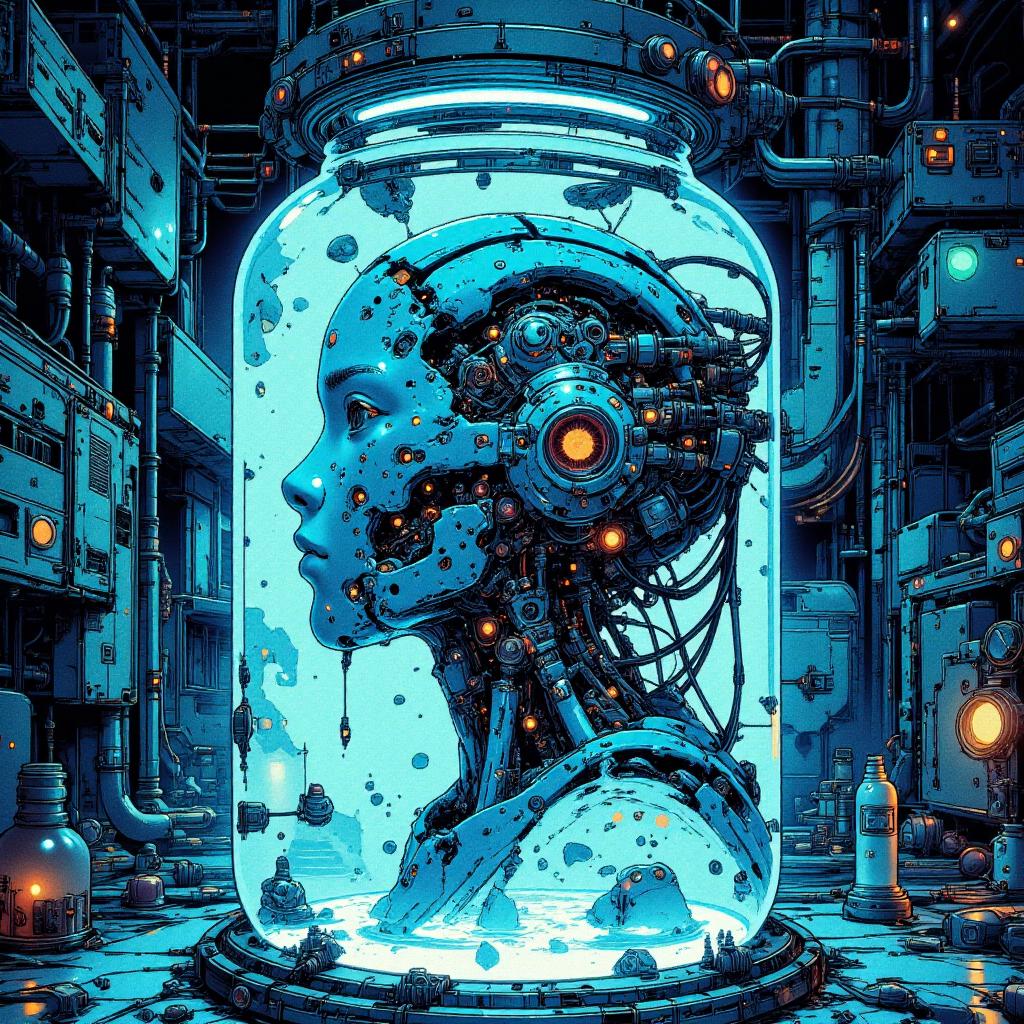
Technologies
Project Overview
Developed a financial assistant chatbot that combines LLM capabilities with real-time stock market data. The system processes natural language queries to provide current stock prices and market insights.
Key Features
- Natural language processing using Groq LLM
- Real-time stock data integration via yfinance
- Type-safe data handling with Pydantic models
- Interactive web interface built with Gradio
- Custom agent configuration with tool integration
Technical Implementation
The project leverages several key technologies to create a robust and user-friendly stock assistant:
Data Model and Type Safety
from pydantic import BaseModel
class StockPriceResult(BaseModel):
symbol: str
price: float
currency: str = "USD"
message: str
LLM Agent Configuration
stock_agent = Agent(
"groq:llama3-groq-70b-8192-tool-use-preview",
result_type=StockPriceResult,
system_prompt="You are a helpful financial assistant specialized in providing stock market information and insights."
)
Stock Data Integration
import yfinance as yf
def get_stock_price(symbol: str) -> StockPriceResult:
ticker = yf.Ticker(symbol)
data = ticker.info
return StockPriceResult(
symbol=symbol,
price=data['regularMarketPrice'],
message=f"Current price for {symbol} is ${data['regularMarketPrice']:.2f}"
)
Gradio Interface
import gradio as gr
def process_query(user_input: str) -> str:
result = stock_agent.run(user_input)
return result.message
interface = gr.Interface(
fn=process_query,
inputs=gr.Textbox(label="Ask about any stock"),
outputs=gr.Textbox(label="Response"),
title="Stock Market Assistant",
description="Get real-time stock information and insights"
)
Key Achievements
- Implemented type-safe data handling using Pydantic models
- Integrated real-time stock market data through yfinance API
- Created an intuitive chat interface using Gradio
- Leveraged Groq’s powerful LLM for natural language understanding
- Developed a modular and extensible architecture
Future Enhancements
- Add historical price analysis capabilities
- Implement portfolio tracking features
- Include market sentiment analysis
- Expand to multiple financial markets
- Add technical indicator calculations
The Stock Assistant demonstrates the power of combining modern LLM capabilities with real-time financial data, creating a practical tool for both casual investors and financial professionals.